{{account.firstName}}
{{lang.index_hello}},{{account.username}} ({{idcsmart_client_level.name}})
ID:{{account.id}}


{{certificationObj.company.certification_company}}
{{lang.index_goAttestation}}
{{lang.finance_custom23}}:
{{certificationObj.company?.certify_id ? certificationObj.company.certify_id : '--'}}
{{certificationObj.company.status === 1 ? certificationObj.company.card_name : certificationObj.person.card_name}}
{{lang.index_goAttestation}}
{{lang.finance_custom24}}:
{{certificationObj.person?.certify_id ? certificationObj.person.certify_id : '--'}}
{{lang.index_text1}}
{{lang.index_text3}}
{{commonData.currency_prefix}}{{account.credit}}
{{lang.index_text2}}
{{lang.finance_text38}}
{{lang.finance_text93}}
{{lang.finance_text94}}
{{lang.finance_text95}}
{{lang.finance_text96}}
{{commonData.currency_prefix}}{{creditData.remaining_amount}}
{{lang.index_text34}}
{{lang.index_text24}}
{{lang.index_text25}}
{{lang.index_text4}}
↑{{Number(account.this_month_consume_percent)}}%
↓{{Number(account.this_month_consume_percent)
*-1}}%
{{commonData.currency_prefix}}{{account.this_month_consume}}
{{lang.index_text5}}
{{commonData.currency_prefix}}{{account.consume}}
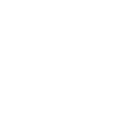
{{lang.index_text6}}
{{account.host_active_num}}
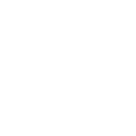
{{lang.index_text7}}
{{account.host_num}}
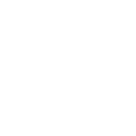
{{lang.index_text8}}
{{account.unpaid_order}}
{{lang.index_text9}}
{{lang.index_text10}} | {{lang.index_text12}} | {{lang.index_text13}} | {{lang.invoice_text139}} |
{{item.product_name}} | {{item.name}} | {{item.due_time | formateTime}} | {{item.client_notes}} |
{{lang.index_text14}}
{{lang.index_text15}}
{{lang.referral_title1}}

{{lang.referral_title6}}
{{lang.referral_text15}}
{{lang.referral_text16}}

{{promoterData.url}}
{{lang.referral_btn2}}
{{commonData.currency_prefix}}{{promoterData.withdrawable_amount}}
{{lang.referral_title2}}

{{commonData.currency_prefix}}{{promoterData.pending_amount}}
{{lang.referral_title4}}


{{lang.index_text17}}
{{lang.index_text18}}
{{lang.index_text28}}
{{lang.index_text21}}
{{lang.index_text22}}
···
{{item.status}}
#{{item.ticket_num}} - {{item.title}}
{{item.name}}
{{lang.index_text23}}
···